728x90
728x90
- 그래프 구성요소
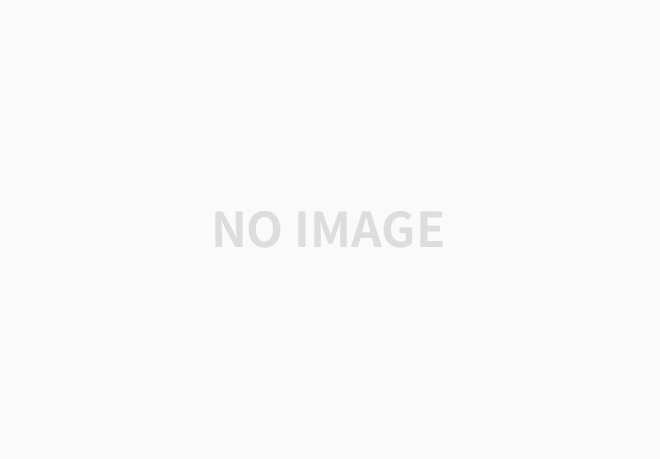
- Figure: 그림 전체
- Axes: 그림 내부의 좌표측 혹은 개별 그림
- Line: 선 그래프에서의 선
- Markers: 점 그래프에서 점
- Legned: 범례
- Grid: 격자
- Title: 제목
- Spines: 윤곽선
- X axis label: X축 라벨
- Y axis lable: Y축 라벨
- Major tick: 메인 눈금
- Major tick label: 메인 눈금 라벨
- Minor tick: 서브 눈금
- Minor tick label: 서브 눈금 라벨
- Matplotlib을 이용한 그래프 그리기
import seaborn as sns
import matplotlib.pyplot as plt
df = sns.load_dataset('penguins')
plt.scatter(df['flipper_length_mm'], df['body_mass_g']) # x축이 flipper_length_mm, y축이 body_mass_g인 산점도 그래프
df_group = df.groupby('species')['body_mass_g'].mean().reset_index() # species별 평균 body_mass_g 계산.
# 그래프를 그리기 위해 x축과 y축이 필요하므로 reset_index()를 통해 인덱스인 species를 열로 변경하는 것입니다.
plt.bar(df_group['species'], height = df_group['body_mass_g']) # bar 그래프에서는 y 대신에 height 입력
plt.hist(df['body_mass_g'], bins = 30) # 히스토그램. bins는 해당 막대의 영역을 얼마나 채우는지를 결정해주는 변수(The towers or bars of a histogram)
plt.xlabel('Body mass') # x축 라벨
plt.ylabel('Count') # y축 라벨
plt.title('Distribution of Penguin's mass') # 제목. matplotlib은 한글 폰트를 기본적으로 지원하지 않음.
# 한글 제목을 쓰고 싶다면 plt.rc('font', family = 'Malgun Gothic') 과 같이 한글을 인식하는 폰트로 바꾸어주어야 합니다.
# spyder 등을 이용해서 코딩할 때 하나씩 적용된 결과만 나온다면 동시에 선택해서 실행해야 합니다.
- plt.plot(x축값, y축값): 선 그래프
- Matplotlib stateless API / Matplotlib stateful API
처음엥는 stateless 방법만 존재했으나, 더 편리한 사용을 위해 wrapper 모듈인 pyplot이 개발이 되어 stateful 방법을 사용할 수 있음.
1. stateless API (objected-based)
내가 지정한 figure, 내가 지정한 axes에 그림을 그리는 방법.
figure와 axes를 직접 만들어야 하고, 이는 객체지향적인 특징을 가지고 있다고 할 수 있습니다.
보다 정교하게 그래프를 표현할 때 사용합니다.
import seaborn as. ns
import matplotlib.pyplot as plt
df = sns.load_dataset('penguins')
fig, axes =. lt.subplots(2, 1, figsize = (10, 6)) # (2,1)은 2행 1열로 2개의 axes 객체를 만들라는 뜻. 가로 10, 세로 6인 figure
axes[0].scatter(df['flipper_length_mm'], df['body_mass_g']) # 1번째 axes에 산점도 그리기
axes[0].set_xlabel('날개 길이(mm)')
axes[0].set_ylabel('몸무게(g)')
axes[0].set_title('날개와 몸무게 간의 관계')
axes[1].hist(df['body_mass_g'], bins = 30) # 2번째 axes에 히스토그램
axes[1].set_xlabel('Body Mass')
axes[1].set_ylabel('Count')
axes[1]/set_title('펭귄의 몸무게 분포')
plt.subplot_adjust(left = 0.1,
right = 0.95,
bottom = 0.1,
top = 0.95,
wspace = 0.5,
hspace = 0.5) # subplots_adjust()는 subplot들의 여백 조정
2. stateful API(state-based)
현재의 figure, 현재의 axes에 그림을 그리는 방법
현재의 figure와 axes를 자동으로 찾아 그곳에 그래프를 나타냅니다.
그래프를 간단히 표현할 때 사용합니다.
import seaborn as. ns
import matplotlib.pyplot as plt
df = sns.load_dataset('penguins')
plt.figure(figsize = (10, 6))
plt.subplot(2, 1, 1) # figure 내에 2행 1열의 axes를 만들며 1번째 axes를 현재 axes로 설정해서 자동으로 첫 번쨰 axes에 그려짐
plt.scatter(df['flipper_length_mm'], df['body_mass_g'])
plt.set_xlabel('날개 길이(mm)')
plt.set_ylabel('몸무게(g)')
plt.set_title('날개와 몸무게 간의 관계')
plt.subplot(2, 1, 2) # 2번째 axes에 그리겠다.
plt.hist(df['body_mass_g'], bins = 30) # 1번째 axes에 히스토그램
plt.set_xlabel('Body Mass')
plt.set_ylabel('Count')
plt/set_title('펭귄의 몸무게 분포')
plt.subplot_adjust(left = 0.1,
right = 0.95,
bottom = 0.1,
top = 0.95,
wspace = 0.5,
hspace = 0.5) # subplots_adjust()는 subplot들의 여백 조정
- Pandas를 이용한 그래프 그리기
- line: 선 그래프
- bar: 수직 막대 그래프
- barh: 수평 막대 그래프
- hist: 히스토그램
- box: 박스 플롯
- kde: 커널 밀도 그래프
- area: 면적 그래프
- pie: 파이 그래프
- scatter: 산점도 그래프
- hexbin: 고밀도 산점도 그래프
import seaborn as sns
df = sns.load_dataset('diamonds')
import pandas as pd
import matplotlib.pyplot as plt
plt.rc('font', family='Malgun Gothic')
# 데이터프레임.plot()을 해주면 데이터프레임 객체를 그림으로 나타냄
df.plot.scatter(x='carat', y='price', figsize = (10, 6), title = '캐럿과 가격 간의 관계')
df.plot.scatter(x = 'carat', y = 'price', c = 'cut', cmap = 'Set2') # c = 'cut'은 cut별로 색깔을 다르게. cmap은 파레트
df['price'].plot.hist(bins = 20) # 히스토그램
df.groupby('color')['carat'].mean().plot.bar() # 데이터 분석 및 시각화를 한 번에
- Seaborn
matplotlib보다 더 화려하고 복잡한 그래프를 표현할 수 있다.
import seaborn as sns
df = sns.load_dataset('titanic')
sns.scatterplot(data = df, x = 'age', y = 'fare', hue = 'class', style = 'class') # 산점도 그래프. hue는 색깔(여기서는 class별로 색깔 다르게). style은 모양
df_pivot =. df.pivot_table(index='class',
columns='sex',
values='survived',
aggfunc='mean')
sns.heatmap(df_pivot, annot = True, cmap = 'coolwarm') # 히트맵 그리기. annot = True를 입력하면 값이 표현됨. cmap은 파레트. coolwarm은 낮으면 파란색, 높으면 빨간색
- figure-level: matplotlib과 별개로 seaborn의 figure를 만들어 그곳에 그래프를 나타냅니다. 따라서 figure-level 함수를 사용할 경우 facetgrid(seaborn의 figure)를 통해 레이아웃을 변경하고, 여러 개의 그래프를 나타낼 수 있습니다.
- axes-level: matplotlib의 axes에 그래프를 나타냅니다.
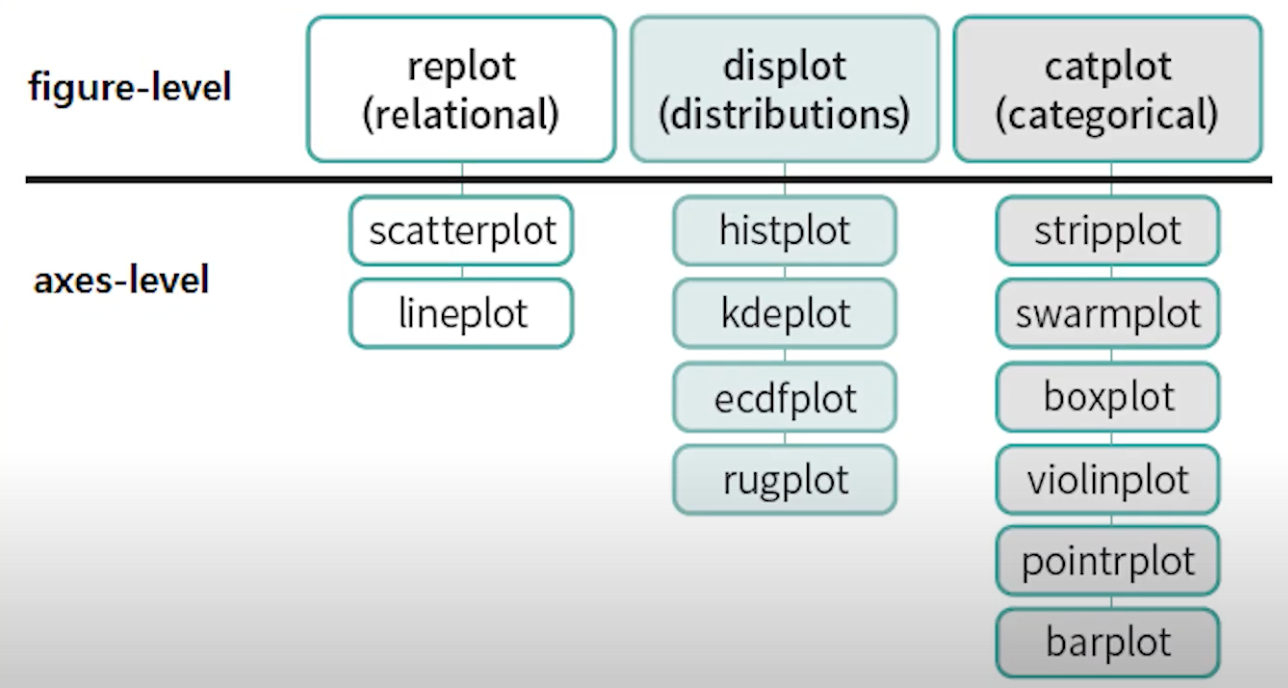
728x90
300x250
'Programming Language > Python' 카테고리의 다른 글
[Python] Python과 SQL 연결 (0) | 2023.12.29 |
---|---|
[Python] ValueError: invalid literal for int() with base 10: '1.70 (0) | 2023.12.29 |
[Python] Pandas package -2 (0) | 2023.12.29 |
[Python] Pandas package - 1 (0) | 2023.12.29 |
[Python] Mac How to fix ModuleNotFoundError: No module named 'Crypto' (1) | 2023.12.29 |