728x90
728x90
https://leetcode.com/problems/merge-k-sorted-lists/
문제
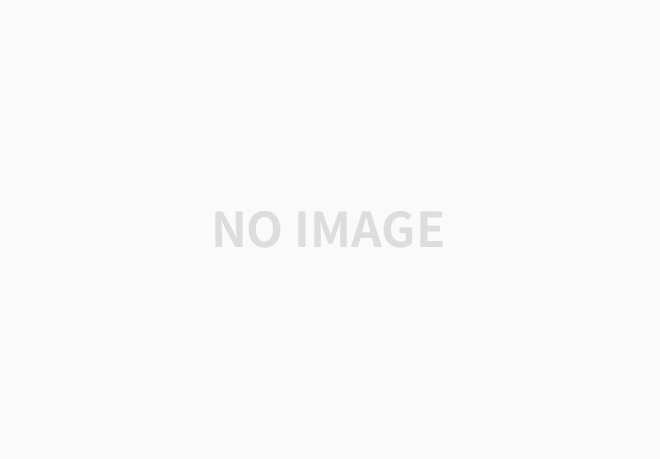
문제 분석
- priority queue를 이용해서 정리한 뒤, 요소를 하나씩 빼서 연결시키면 됩니다.
- 어떤 방식으로든지 정렬을 해서 하나씩 뽑아서 넣으면 됩니다.
풀이
class Solution {
public:
ListNode* mergeKLists(vector<ListNode*>& lists) {
if (lists.empty()){
return nullptr;
}
auto compare = [](ListNode* a, ListNode* b){
return a->val > b->val;
};
std::priority_queue<ListNode*, std::vector<ListNode*>, decltype(compare)> pq(compare);
for(ListNode* list: lists){
if (list != nullptr){
pq.push(list);
}
}
ListNode dummy;
ListNode* tail = &dummy;
while(!pq.empty()){
ListNode* smallest = pq.top();
pq.pop();
tail->next = smallest;
tail = tail->next;
if (smallest->next != nullptr){
pq.push(smallest->next);
}
}
return dummy.next;
}
};
728x90
300x250
'Problem Solving > LeetCode' 카테고리의 다른 글
[LeetCode] C++ 200. Number of Islands (0) | 2024.09.01 |
---|---|
[LeetCode] C++ 706. Design HashMap (0) | 2024.08.26 |
[LeetCode] C++ 739. Daily Temperatures (0) | 2024.08.19 |
[LeetCode] C++ 316. Remove Duplicate Letters (0) | 2024.08.18 |
[LeetCode] C++ 20. Valid Parentheses (0) | 2024.08.18 |