728x90
728x90
Traversal
- The process of visiting each node in a graph.
- How to visit all nodes once?
- Depth-first search(DFS)
- Breadth-first search(BFS)
Depth-First Search(DFS)
- Keep moving until there is no more possible block.
- Go back the previous step and move other unvisited block.
- Algorithm of DFS
- Visit the start vertex.
- Visit one of unvisited vertices neighboring to the start vertex.
- Repeat step 2 until there is no more unvisited vertex.
- If there is no more unvisited vertex, go back one step and visit another unvisited vertex.
- DFS is implemented with the stack.
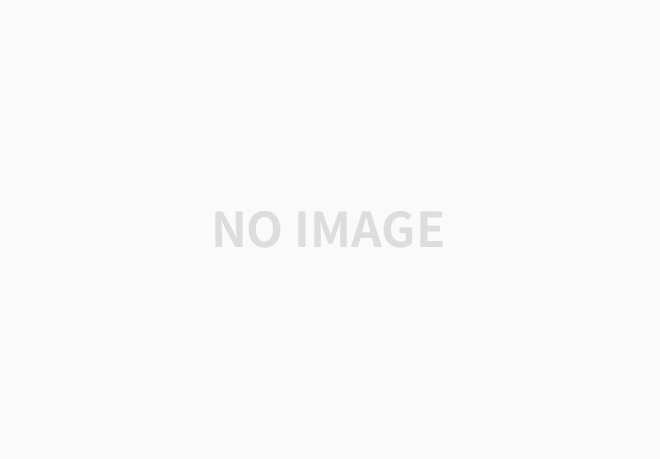
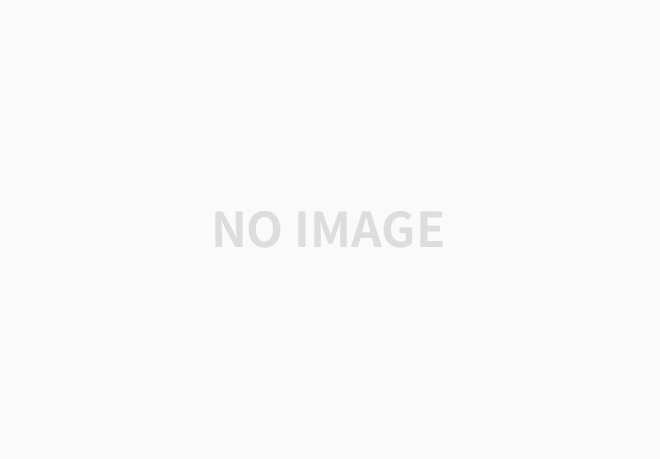
void DFS(Graph* pgraph)
{
Stack stack;
bool* visited = (bool *)malloc(sizeof(bool)* pgraph->num);
for (int i = 0; i < pgraph->num; i++)
visited[i] = false // Make all vertices unvisited.
InitStack(&stack);
Push(&stack, 0); // Push the initial vertex;
while (!IsSEmpty(&stack){
GNode* cur;
int vtx = SPeek(&stack);
Pop(&stack);
//skip if the vertex has been visited.
if (visited[vtx]) continue;
else{
visited[vtx] = true;
printf("%d ", vtx);
}
// Push the vertex if it has been unvisited.
cur = pgraph->heads[vtx]->next;
while (cur != NULL) {
if(!visited[cur->id])
Push(&stack, cur->id);
cur = cur->next;
}
}
}
Breadth-First Search(BFS)
- Keep moving step-by-step for all possible blocks.
- Algorithm of BFS
- Visit the start vertex.
- Visit all unvisited vertices neighboring to the start vertex.
- Repeat step 2 until there is no more unvisited vertex.
- BFS is implemented with the queue.
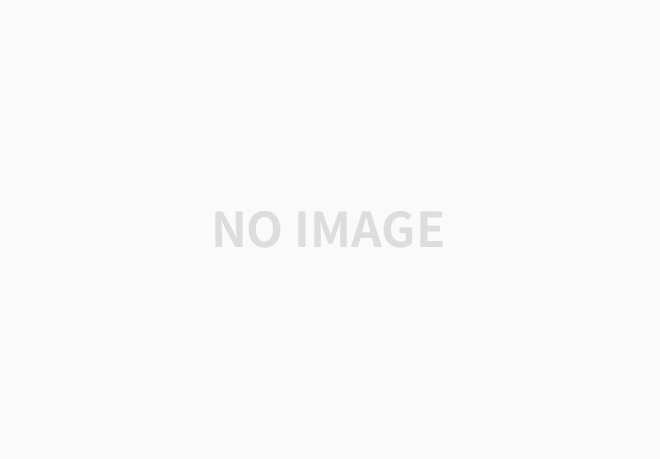
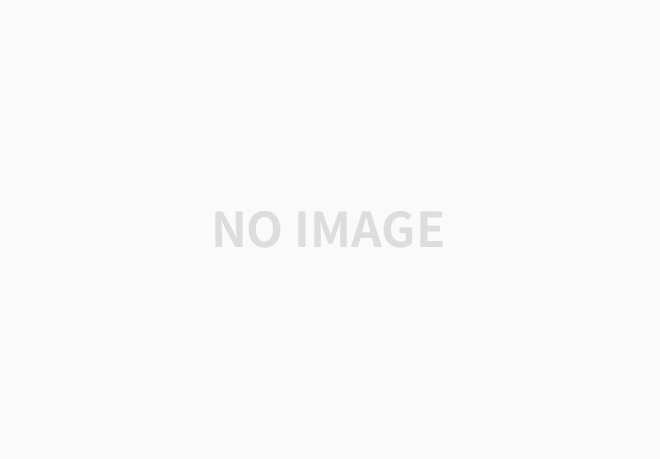
void BFS(Graph* pgraph)
{
Queue queue;
bool* visited = (bool *)malloc(sizeof(bool)* pgraph->num);
for (int i = 0; i < pgraph->num; i++)
visited[i] = false; // Make all vertices unvisited.
InitQueue(&queue);
EnQueue(&queue, 0); // Enqueue the initial vertex;
while(!IsQEmpty(&queue)){
GNode* cur;
int vtx = QPeek(&queue);
DeQueue(&queue);
// Skip if the vertex has been visited.
if (visited[vtx]) continue;
else {
visited[vtx] = true;
printf("%d ", vtx);
}
// Enqueue the vertex if it has been unvisited.
cur = pgraph->heads[vtx]->next;
while (cur != NULL){
if(!visited[cur->id])
EnQueue(&queue, cur->id);
cur = cur->next;
}
}
}
728x90
300x250
'Computer Science > Algorithm' 카테고리의 다른 글
[Algorithm] Shell sort (0) | 2023.12.26 |
---|---|
[Algorithm] Bubble sort (0) | 2023.12.26 |
[Algorithm] Selection sort (0) | 2023.12.26 |
[Algorithm] Sorting algorithm (0) | 2023.12.26 |
[Algorithm] Priority Queue and Heap (1) | 2023.12.26 |